Automation and repetition are ubiquitous concepts in programming. Imagine having to perform the same action hundreds or even thousands of times. This would not only be tedious, but also a source of errors. Programming languages such as Python offer powerful tools for managing these repetitions: loops.
Whether you’re browsing a list of data, repeating an operation until a condition is met, or even generating sequences, loops are of paramount importance.
Â
💡Related articles:
The "for" loop
1. Basic concept
Its syntax is simple and intuitive:
for element in sequence:
# block of code to execute for each element
For example, to display each letter of a string :
for letter in "Python":
print(letter)
2. Use with the range() function
for i in range(5):
print(i)
3. Browse lists
noms = ["Alice", "Bob", "Charlie"]
for nom in noms:
print(nom)
4. Nested loops
for i in range(1, 4):
for j in range(1, 4):
print(f"{i} x {j} = {i*j}")
Nested loops can be powerful, but should be used with caution to avoid excessive complexity.
The "while" loop
1. Basic concept
Its syntax is as follows:
while condition:
# block of code to be executed as long as the condition is true
For example, to display numbers from 0 to 4 :
i = 0
while i < 5:
print(i)
i += 1
Note the importance of incrementing variable i at each iteration to avoid an endless loop.
2. Precautions
- Make sure you have a condition that will become false at some point.
- Check conditions and control variables regularly during the development phase.
Let’s take an example of an infinite loop. Suppose we want to double the value of a variable until it reaches (or exceeds) a certain threshold.
limit = 100
value = 1
while value < limit:
print(value)
# Forgot to increment or modify value
# Instead of doubling the value as planned, we forgot this step
# value *= 2
3. Practical use
password = "secret
input = ""
while input != password:
input = input("Enter password: ")
print("Access granted.")
Flow control in loops
Python offers several tools for managing the flow of execution within loops, allowing greater flexibility.
1. Break and continue instructions
- break : Exit the current loop immediately. Execution resumes at the code block following the loop.
Example: Find the first number divisible by 7 in a list.
numbers = [3, 5, 8, 12, 14, 18]
for n in numbers:
if n % 7 == 0:
print(f "The first number divisible by 7 is {n}.")
break
- continue : Interrupts the current iteration and proceeds to the next, without exiting the loop.
Example: Display all numbers except those divisible by 3.
for i in range(10):
if i % 3 == 0:
continue
print(i)
2. The else clause with loops
n = 17
for i in range(2, n):
if n % i == 0:
print(f"{n} is not a prime number.")
break
else:
print(f"{n} is a prime number.")
Tips and best practices
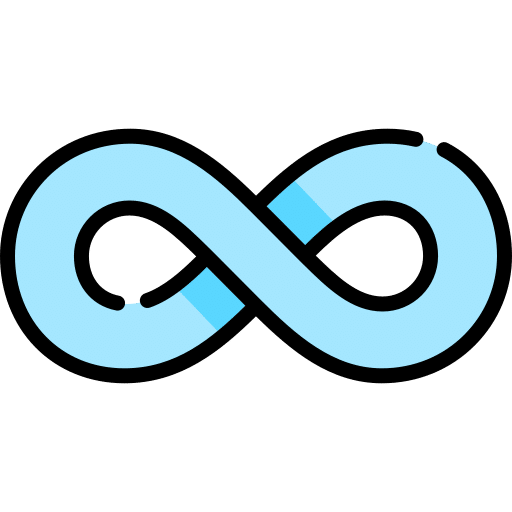
Infinite loops
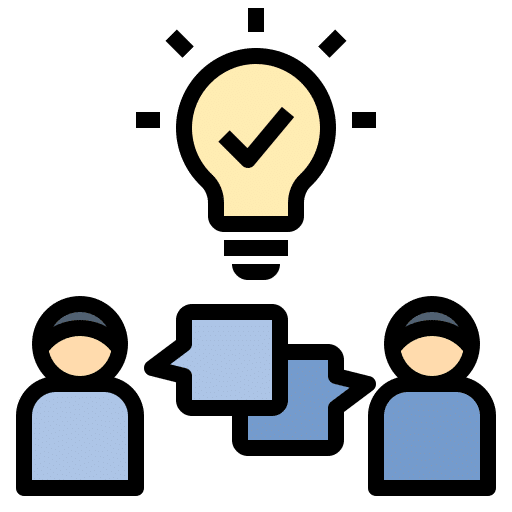
List comprehension
A Python feature to create lists in a concise and elegant way. Instead of a loopfor, use list comprehension..
Example : Create a list of the squares of the numbers from 0 to 9.
squares = [x**2 for x in range(10)]
Â
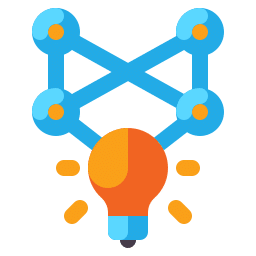
Limit the depth of interlocking loops
Avoid too many overlapping loops, as they complicate legibility. For more than three levels, consider other solutions.
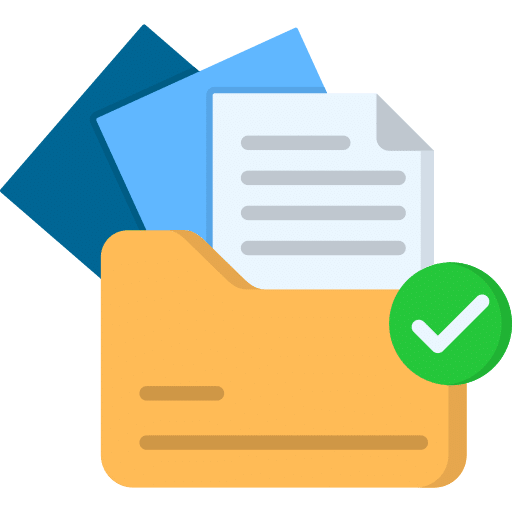
Document
A comment clarifies the purpose of the loop, especially for complex loops.
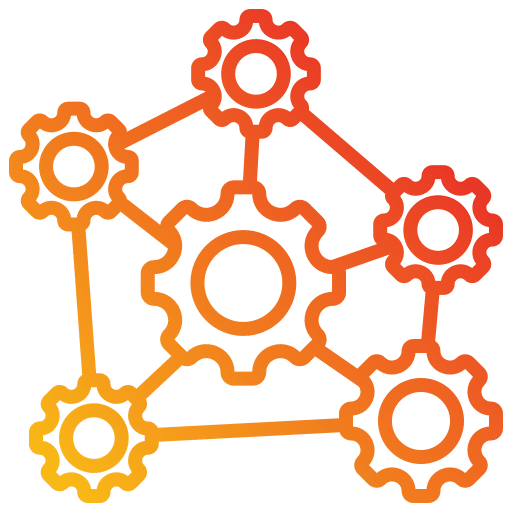
Beware of complexity
Structure your loops wisely to optimize performance. Avoid costly in-loop operations.
Conclusion
Mastering loops is crucial for any Python developer. They offer the power to automate repetitive tasks, making code more efficient and concise. By following best practices and understanding the subtleties, you’ll optimize your programs while avoiding common pitfalls.